Phone Input (AI Assistant)
This page provides information on using the Phone Number Input widget (available in AI Assistant Apps), which allows you to capture and validate phone number user inputs.
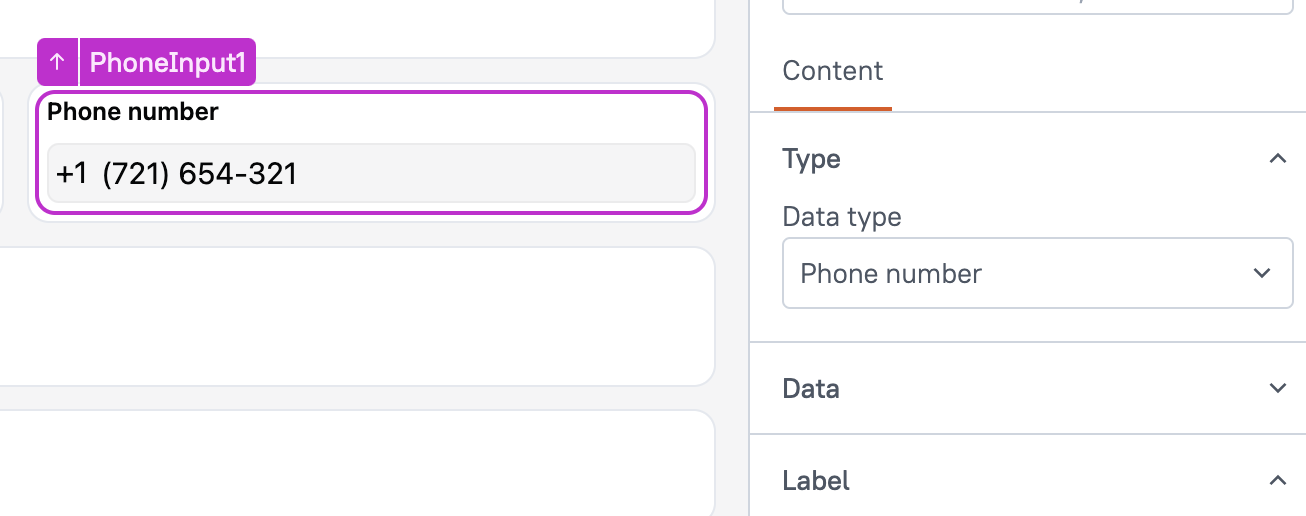
Content properties
These properties are customizable options present in the property pane of the widget, allowing users to modify the widget according to their preferences.
Type
Data type string
The Data Type property defines the type of input for the widget. For the Phone Number Input widget, the Data Type is set to Phone Number by default. If you change the data type, the widget’s properties and behavior adjust accordingly to match the selected input type.
Options:
- Single-line text: Accepts a single line of text, such as names or titles. Additional text beyond one line is not displayed.
- Multi-line text: Allows multiple lines of text, ideal for longer entries like comments or descriptions.
- Number: Accepts only numeric values.
- Password: Masks input for sensitive information such as passwords or pins.
- Email: Validates and accepts text in email format.
- Phone number: Accepts phone numbers, often formatted with country code and dashes.
- Currency: Accepts numeric input displayed in currency format.
- Date: Accepts date input, with a datepicker for selection.
Data
Value string
Defines the initial value displayed in the widget when it loads. This value serves as the default input until the user modifies it. To dynamically populate the field with a value from a table, you can reference a selected row's data, such as a phone number from a database or table. For example:
{{Table1.selectedRow.phoneNumber}}
Default country code
The Default Country Code property allows users to select a country from a dropdown or manually enter a country code (e.g., +256
). You can dynamically update the country code based on the user's selection or input.
{{CountryDropdown.selectedOptionValue === "Uganda" ? "+256" : "+1"}}
Label
The Label property is a group of customizable settings that define the main text displayed on the widget.
Text string
Specifies the text displayed as the label on the widget. This can be used to indicate the purpose or function of the widget.
Validation
The Validation properties ensure that the input provided by users meets specific criteria. These settings allow you to define rules and conditions to validate the data entered into the widget
Required boolean
This validation feature allows you to designate the Input as a mandatory field. For instance, when the Input is placed within a Form widget, enabling the Required property ensures that the Form's submit button remains disabled until the Input has some value.
Regex string
The Regex property, short for Regular Expression, enables you to apply custom validations on user input by defining specific constraints using regular expressions. If the user enters a value that does not adhere to the specified pattern, the widget displays an error message indicating "invalid input"
.
Examples:
//Regex for phone number with country code
^\+(\d{1,3})\s(\d{1,4})\s(\d{3})\s(\d{4})$
Valid boolean
Allows you to define custom validation rules and error messages to guide users when their input doesn't meet required criteria.
For instance, you can use this property to validate a Phone Number Input field, ensuring the number follows a valid format, such as a 10-digit phone number.
Example:
{{
/^\d{10}$/.test(PhoneNumberInput.text)
}}
Error Message string
Allows customization of the error message displayed when the user enters an incorrect value. By default, the input widget shows a generic "invalid input"
message.
Example: If you want to validate a Phone Number Input, ensuring it contains exactly 10 digits, you can use the following code in the Error message property.
//Valid property
{{/^\d{10}$/.test(PhoneNumberInput.text) ? true : false}}
// Error message property
{{!/^\d{10}$/.test(PhoneNumberInput.text) ? "Error: The phone number must be exactly 10 digits" : ""}}
This ensures that the user is prompted with a custom error message when the number entered is outside the specified range of 10 to 100.
General
General properties are essential configurations that provide overall control over the widget's behavior and appearance.
Tooltip string
Allows you to add a short message or hint that provides additional information to guide the user. A ? icon appears near the label text, and when the user hovers over it, the tooltip message is displayed.
Placeholder string
Allows you to set the placeholder text displayed within the input box. This can be used to provide a hint or example value to the user, guiding them on the expected format or content of the input.
Visible boolean
Controls the visibility of the widget. If you turn off this property, the widget would not be visible in View Mode. Additionally, you can use JavaScript by clicking on JS next to the Visible property to conditionally control the widget's visibility. The default value for the property is true
.
For example, if you want to make the widget visible only when the user selects "Yes" from a Select widget, you can use the following JavaScript expression:
{{Select1.selectedOptionValue === "Yes"}}
Disabled boolean
Prevents users from selecting the widget. Even though the widget remains visible, user input is not permitted. Additionally, you can use JavaScript by clicking on JS next to the Disabled property to control the widget's disable state conditionally. The default value for the property is false
.
For example, if you want to allow only a specific user to fill the input, you can use the following JavaScript expression:
{{appsmith.user.email=="john@appsmith.com"?false:true}}
Readonly boolean
When enabled, the widget displays the value from the Value property and prevents user input. This also disables Validation and Event properties, ensuring the value cannot be modified. It functions similarly to the Key-Value widget. Readonly is not available when the Data Type is set to Date.
Animate Loading boolean
This property controls whether the widget is displayed with a loading animation. When enabled, the widget shows a skeletal animation during the loading process. Additionally, you can control it through JavaScript by clicking on the JS
next to the property. The default value for the property is true
.
Auto Focus boolean
When enabled, automatically places the user's cursor in the input box upon page load, directing their attention to the input field for immediate interaction.
Events
Events are properties that allow you to define actions or responses based on user interactions or widget state changes.
When the event is triggered, these event handlers can execute queries, JS functions, or other supported actions.
onTextChanged
Specifies the actions to be executed when the input value is modified. This event is triggered every time the user changes the text in the input field.
onFocus
Specifies the actions to be executed when the input area is focused.
onBlur
Specifies the actions to be executed when the input field loses focus. This event is typically used for form validation, submitting data, or updating widget.
onSubmit
Specifies the actions to be executed when the input is submitted using the ENTER
key. This event is often used in forms or search fields where the user expects the input to trigger an action upon submission.
Reset on submit
Clears the input value after submission. This ensures that the field is reset to its default state, which is useful for clearing out forms after data is submitted or when starting a new entry.
Reference properties
Reference properties are properties that are not available in the property pane but can be accessed using the dot operator in other widgets or JavaScript functions. They provide additional information or allow interaction with the widget programmatically. For instance, to get the visibility status, you can use PhoneInput1.isVisible
.
parsedText string
The parsedText
property retrieves the input value of the widget.
Example:
{{PhoneInput1.text}}
isValid boolean
The isValid
property indicates the validation status of a widget, providing information on whether the widget's current value is considered valid or not.
Example:
{{PhoneInput1.isValid}}
isReadOnly boolean
The isReadOnly
property indicates the read-only state of a widget, with true
indicating that the widget is in read-only mode and cannot be edited by the user, while false
allows the user to interact and modify the widget's value.
Example:
{{PhoneInput1.isReadOnly}}
isDisabled boolean
The isDisabled
property reflects the state of the widget's Disabled setting. It is represented by a boolean value, where true indicates that the widget is not available, and false indicates that it is enabled for user interaction.
Example:
{{PhoneInput1.isDisabled}}
isVisible boolean
The isVisible
property indicates the visibility state of a widget, with true indicating it is visible and false indicating it is hidden.
Example:
{{PhoneInput1.isVisible}}
Methods
Widget property setters enable you to modify the values of widget properties at runtime, eliminating the need to manually update properties in the editor.
These methods are asynchronous and return a Promise. You can use the .then()
block to ensure execution and sequencing of subsequent lines of code in Appsmith.
setVisibility (param: boolean): Promise
Sets the visibility of the widget. This method is useful when you want to dynamically show or hide a widget based on certain conditions, such as form steps or user inputs.
Example:
PhoneInput1.setVisibility(true)
setDisabled (param: boolean): Promise
Sets the disabled state of the widget. This method can be used to prevent user interactions with the widget.
Example:
PhoneInput1.setDisabled(false)
Example: If you want to disable an input field for anonymous users, you can use:
if (appsmith.user.isAnonymous) {
PhoneInput1.setDisabled(true) // Disable input for anonymous users
} else {
PhoneInput1.setDisabled(false) // Enable input for logged-in users
}
setValue (param: string): Promise
Allows you to dynamically set the value of the widget. This is useful when you need to populate a field with data, such as pre-filling a form based on user selection or data from a query.
Example:
PhoneInput1.setValue("+256241234567")
setRequired (param: boolean): Promise
Sets whether the widget is required or not. This method can be used to dynamically adjust form validation rules based on specific conditions.
Example:
PhoneInput1.setRequired(true)
setReadOnly (param: boolean): Promise
Sets the read-only state of the widget. This method is useful when you want to prevent the user from editing the widget while still displaying its value. It can be used for scenarios like showing data that should not be modified by the user, or for temporarily disabling editing.
Example:
PhoneInput1.setReadOnly(true)
Example: If you only want the widget to be editable for specific users (e.g., logged-in users), use:
if (appsmith.user.isAnonymous) {
PhoneInput1.setReadOnly(true) // Prevent modification for anonymous users
} else {
PhoneInput1.setReadOnly(false) // Allow modification for logged-in users
}